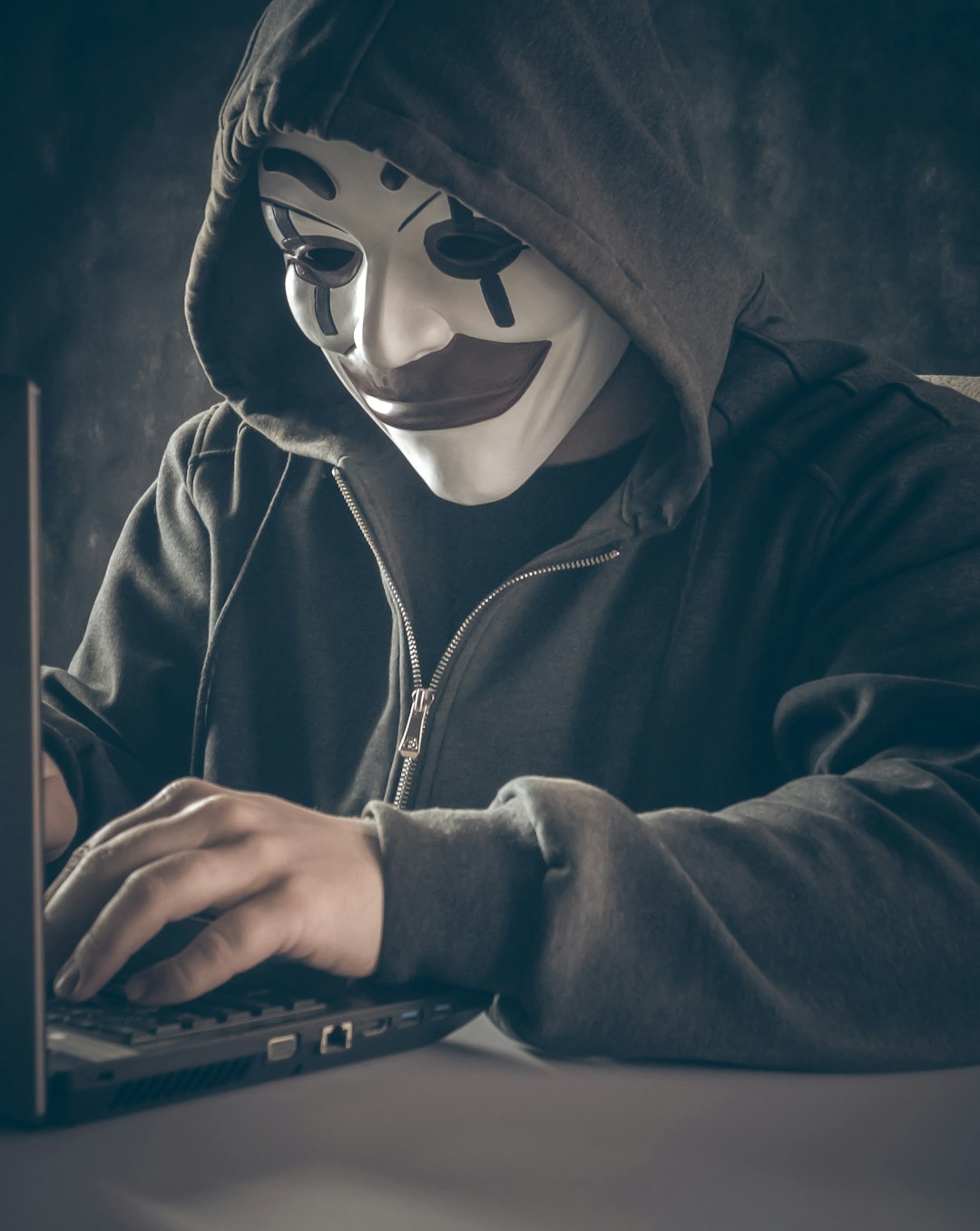
Second-level caching in Hibernate is a powerful feature that can significantly improve application performance by reducing database access. However, a common question among developers is whether it is mandatory to enable this caching mechanism when working with Hibernate.
Understanding Hibernate’s Caching Mechanism
Hibernate provides two levels of caching: first-level and second-level cache. The first-level cache is mandatory and enabled by default, meaning every Hibernate session has its caching mechanism. However, the second-level cache is optional and needs to be explicitly configured.
- First-Level Cache: This cache is associated with a Hibernate session and is used to reduce database access within the session’s scope.
- Second-Level Cache: This cache is shared across multiple sessions and allows for data reuse between transactions, reducing database load.
Is Second-Level Caching Mandatory?
The short answer is no, second-level caching is not mandatory in Hibernate. Hibernate does not require its implementation, and an application can function correctly without it. However, whether or not to enable this cache depends on the specific needs of the application.
When Should You Use Second-Level Caching?
The decision to use second-level caching should be based on application performance considerations. Below are some scenarios where enabling it can be beneficial:
- Frequent Reads: If an application frequently retrieves the same data without many modifications, caching can reduce database queries and enhance response times.
- Low Database Change Rate: If the application’s data does not change often, caching can be highly effective in reducing the load on the database.
- Scalability Requirements: Applications with a large user base making repeated queries can benefit from caching to handle high traffic efficiently.
On the other hand, there are cases where using second-level caching might not be the best option:
- If the application requires immediate data consistency and changes in the database must be reflected instantly.
- For applications with highly transactional data where caching may lead to stale data being read.
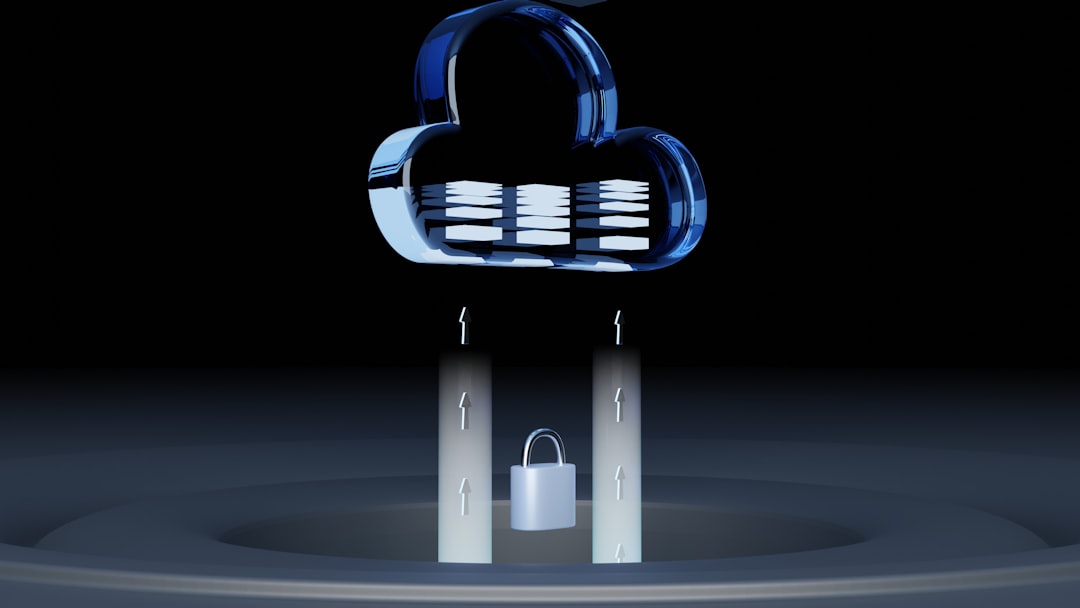
How to Enable Second-Level Cache in Hibernate
To enable second-level caching in Hibernate, you need to integrate a caching provider. Hibernate supports multiple cache providers, including:
- Ehcache
- Infinispan
- Hazelcast
Steps to Configure Second-Level Cache
- Enable Hibernate Second-Level Cache: You can enable it by setting the property
hibernate.cache.use_second_level_cache=true
. - Choose a Caching Provider: Choose from providers like Ehcache or Infinispan and include the necessary dependencies in your application.
- Annotate Entities for Caching: Use the
@Cache
annotation on relevant entities to specify caching strategy.
For example, with Ehcache, you would configure caching in ehcache.xml
and annotate entities as follows:
@Cacheable @Cache(usage = CacheConcurrencyStrategy.READ_WRITE) @Entity public class Product { ... }
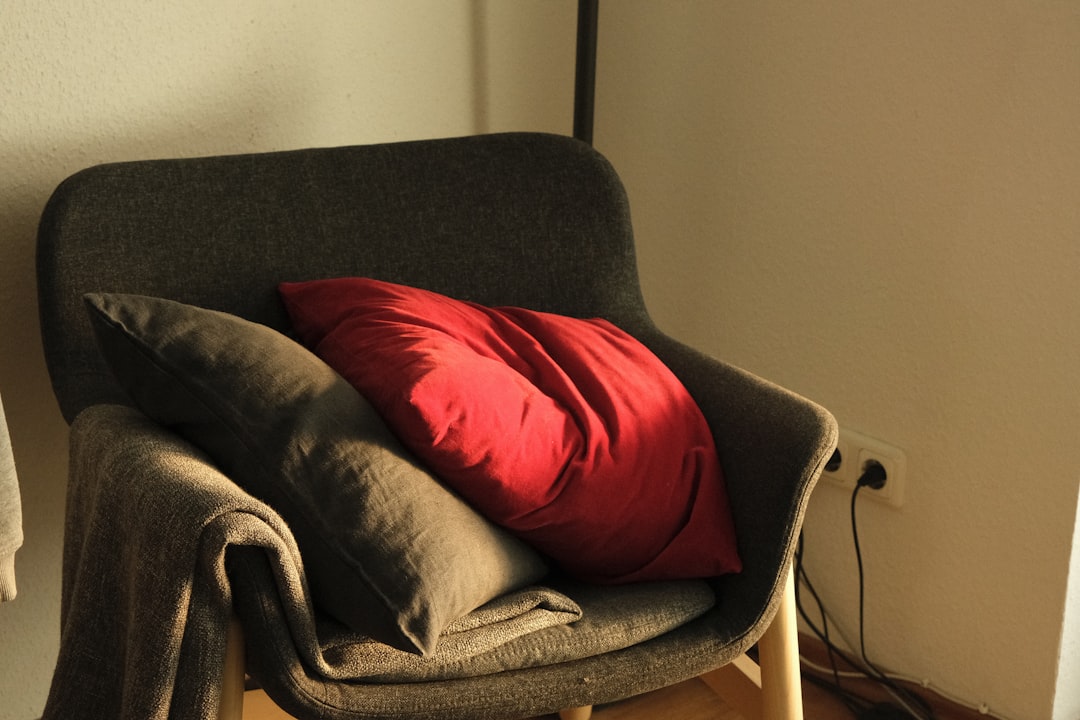
Potential Challenges of Using Second-Level Caching
While caching can improve performance, it also introduces additional complexities:
- Data Inconsistency: Cached data might become outdated if the application does not carefully manage cache refresh mechanisms.
- Increased Memory Usage: Enabling caching requires extra memory, which may not be suitable for applications with limited resources.
- Cache Eviction Policies: Choosing the right eviction policy is crucial to ensure data freshness without unnecessarily invalidating useful information.
Conclusion
Second-level caching in Hibernate is not mandatory. While it can provide significant performance enhancements, not all applications will benefit from it. The decision to implement caching should be based on an application’s access patterns, scalability needs, and available resources.
For applications with frequent read operations on relatively unchanged data, enabling second-level caching can reduce database access times and improve efficiency. However, for transactional applications requiring immediate and consistent database updates, caching might introduce unwanted complexities.
Ultimately, developers should assess whether the trade-offs of implementing second-level caching align with their application’s requirements.